Copilot Chat in Neovim
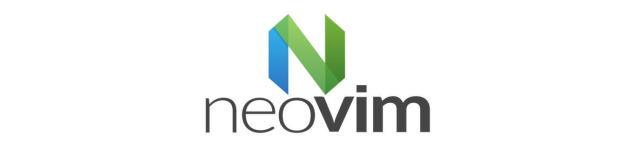
TL;DR
Copilot Chat is feature of Github Copilot that allows you to chat with the AI to get suggestions on how to write code. This feature is available only on VScode and InteliJ IDEs, at the moment. However, you can use it in Neovim with the help of the Copilot Chat plugin.
This plugin uses your Github token to authenticate with the Copilot API and it allows you to chat with the AI in Neovim.
Requirements
- Neovim 0.95 or later.
- A Github account with Copilot subscription.
Installation
To install the plugin with lazyvim use the following lua configuration
return {
{
"CopilotC-Nvim/CopilotChat.nvim",
branch = "canary",
dependencies = {
{ "github/copilot.vim" }, -- or github/copilot.vim
{ "nvim-lua/plenary.nvim" }, -- for curl, log wrapper
{ "nvim-telescope/telescope.nvim" }, -- Use telescope for help actions
},
opts = {
debug = true, -- Enable debugging
show_help = true, -- Show help actions
window = {
layout = "float",
},
auto_follow_cursor = false, -- Don't follow the cursor after getting response
},
config = function(_, opts)
local chat = require("CopilotChat")
local select = require("CopilotChat.select")
-- Use unnamed register for the selection
opts.selection = select.unnamed
-- Override the git prompts message
opts.prompts.Commit = {
prompt = "Write commit message for the change with commitizen convention",
selection = select.gitdiff,
}
opts.prompts.CommitStaged = {
prompt = "Write commit message for the change with commitizen convention",
selection = function(source)
return select.gitdiff(source, true)
end,
}
chat.setup(opts)
vim.api.nvim_create_user_command("CopilotChatVisual", function(args)
chat.ask(args.args, { selection = select.visual })
end, { nargs = "*", range = true })
-- Inline chat with Copilot
vim.api.nvim_create_user_command("CopilotChatInline", function(args)
chat.ask(args.args, {
selection = select.visual,
window = {
layout = "float",
relative = "cursor",
width = 1,
height = 0.4,
row = 1,
},
})
end, { nargs = "*", range = true })
-- Restore CopilotChatBuffer
vim.api.nvim_create_user_command("CopilotChatBuffer", function(args)
chat.ask(args.args, { selection = select.buffer })
end, { nargs = "*", range = true })
end,
event = "VeryLazy",
keys = {
-- Show help actions with telescope
{
"<leader>cch",
function()
local actions = require("CopilotChat.actions")
require("CopilotChat.integrations.telescope").pick(actions.help_actions())
end,
desc = "CopilotChat - Help actions",
},
-- Show prompts actions with telescope
{
"<leader>ccp",
function()
local actions = require("CopilotChat.actions")
require("CopilotChat.integrations.telescope").pick(actions.prompt_actions())
end,
desc = "CopilotChat - Prompt actions",
},
-- Code related commands
{ "<leader>cce", "<cmd>CopilotChatExplain<cr>", desc = "CopilotChat - Explain code" },
{ "<leader>cct", "<cmd>CopilotChatTests<cr>", desc = "CopilotChat - Generate tests" },
{ "<leader>ccr", "<cmd>CopilotChatReview<cr>", desc = "CopilotChat - Review code" },
{ "<leader>ccR", "<cmd>CopilotChatRefactor<cr>", desc = "CopilotChat - Refactor code" },
{ "<leader>ccn", "<cmd>CopilotChatBetterNamings<cr>", desc = "CopilotChat - Better Naming" },
-- Chat with Copilot in visual mode
{
"<leader>ccv",
":CopilotChatVisual",
mode = "x",
desc = "CopilotChat - Open in vertical split",
},
{
"<leader>ccx",
":CopilotChatInline<cr>",
mode = "x",
desc = "CopilotChat - Inline chat",
},
-- Custom input for CopilotChat
{
"<leader>cci",
function()
local input = vim.fn.input("Ask Copilot: ")
if input ~= "" then
vim.cmd("CopilotChat " .. input)
end
end,
desc = "CopilotChat - Ask input",
},
-- Generate commit message based on the git diff
{
"<leader>ccm",
"<cmd>CopilotChatCommit<cr>",
desc = "CopilotChat - Generate commit message for all changes",
},
{
"<leader>ccM",
"<cmd>CopilotChatCommitStaged<cr>",
desc = "CopilotChat - Generate commit message for staged changes",
},
-- Quick chat with Copilot
{
"<leader>ccq",
function()
local input = vim.fn.input("Quick Chat: ")
if input ~= "" then
vim.cmd("CopilotChatBuffer " .. input)
end
end,
desc = "CopilotChat - Quick chat",
},
-- Debug
{ "<leader>ccd", "<cmd>CopilotChatDebugInfo<cr>", desc = "CopilotChat - Debug Info" },
-- Fix the issue with diagnostic
{ "<leader>ccf", "<cmd>CopilotChatFixDiagnostic<cr>", desc = "CopilotChat - Fix Diagnostic" },
-- Clear buffer and chat history
{ "<leader>ccl", "<cmd>CopilotChatReset<cr>", desc = "CopilotChat - Clear buffer and chat history" },
-- Toggle Copilot Chat Vsplit
{ "<leader>ccv", "<cmd>CopilotChatToggle<cr>", desc = "CopilotChat - Toggle Vsplit" },
},
},
}
Then, restart Neovim and lazy vim will install the plugin.
Open Copilot Chat
To open the Copilot Chat, use the following key binding:
<leader>ccv
with the the previus configuration the chat will open in a floating window.
Use Copilot Chat with pre-defined actions
The plugin comes with pre-defined actions that you can use to chat with Copilot. The following are the available actions:
To use this feature, first selecte the code or text that you want to use in the chat and "y" (Yanked), then use the following key binding:
<leader>ccp
For this feature you need to have the Telescope plugin installed.
Features
Inline chat with Copilot:
First select the code you want to use in the chat and on visual mode use the following key binding:
<leader>ccx
Explain code:
First select the code you want to explain and y
(Yanked), then use the following key binding:
<leader>cce
Unit Test code:
First select the code you want to test and y
(Yanked), then use the following key binding:
<leader>cct
Review code:
First select the code you want to review and y
(Yanked), then use the following key binding:
<leader>ccr
Refactor code:
First select the code you want to refactor and y
(Yanked), then use the following key binding:
<leader>ccR
Better Naming:
First select the code you want to change the names and y
(Yanked), then use the following key binding:
<leader>ccn