Django models
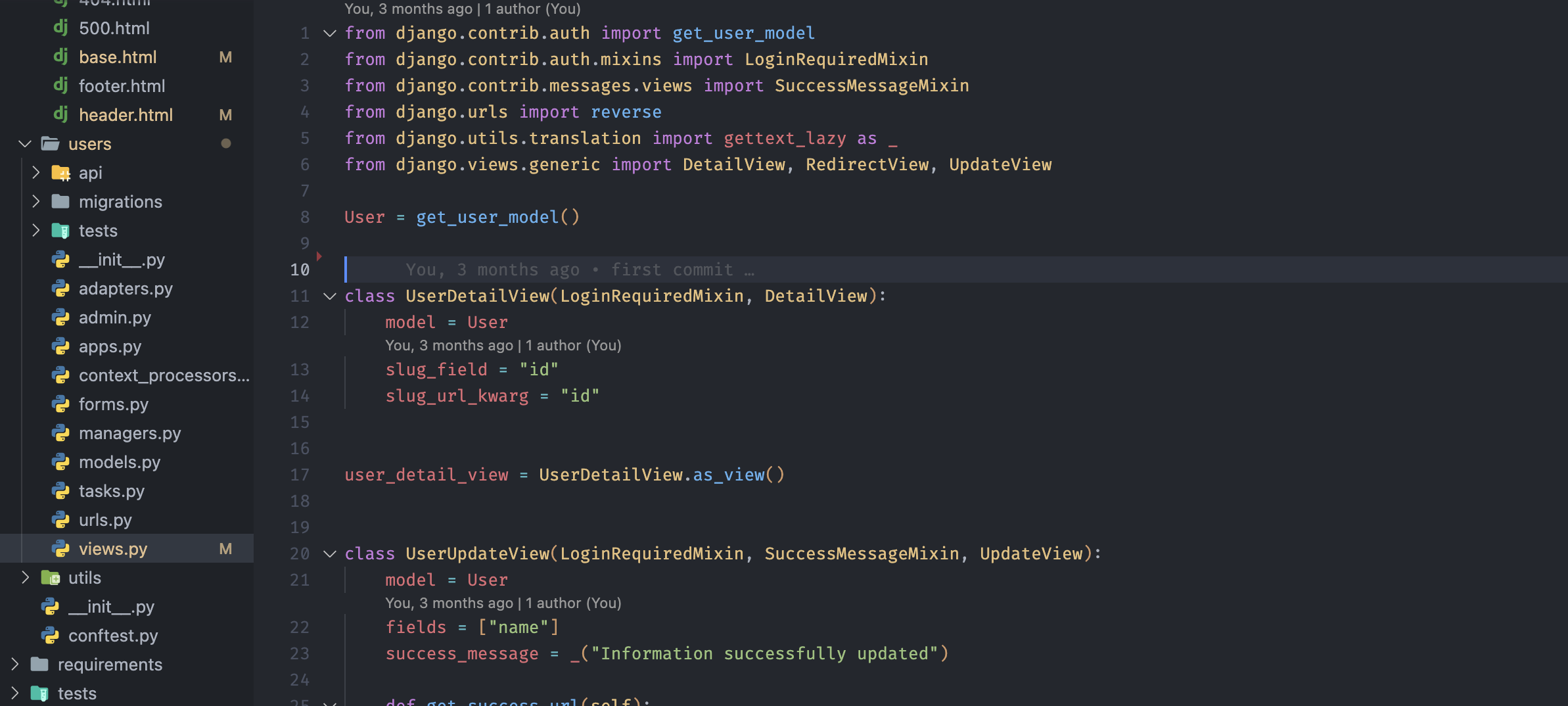
This post's to remain me what are the different types of models that I can use in a Django app.
Models
Django uses an ORM "Object-related Mapping" to interact with the database. That means a Python Class "model" represent a table of the database. But these models could have extra functionalities.
Example:
Model :
from django.db import models
class Person(models.Model):
name = models.CharField()
birthday = models.DateField()
Table:
Person | ||
---|---|---|
name | birthday | |
Emily Glass | 25/09/1986 | |
Tom Example | 30/01/1991 |
Model inheritance
There are 3 types of model inheritances:
- Proxy Models
- Multi-tale Models
- Abstract Models
Proxy Models
Proxy models are Python class that inherits from a model to add extra functionality without creating a new table in the database.[1]
Example:
from django.db import models
class Person(models.Model):
first_name = models.CharField()
last_name = models.CharField()
class MyPerson(Person):
class Meta:
proxy = True
def say_something(self, msg):
print(msg)
Multi-table Models
Multi-table model inheritance is created by class inherits from an existing model. Django translate this in a new table with a One To One relation with the father model.
from django.db import models
class Place(models.Model):
name = models.CharField()
address = models.CharField()
class Restaurant(Place):
menu = models.CharField()
Abstract models
Abstract models are base models use to create model more complex. Abstracts models do not represent a table in the database. To create an abstract model we need to specify the Meta class inside the class model.
Example:
from django.db import models
class Person(models.Model):
"""Abstract Class"""
first_name = models.CharField()
last_name = models.CharField()
...
class Meta:
""" Meta Options """
abstract = True
class Student(Person):
school_name = models.CharField()
...
In this case the student table looks like:
Student | ||
---|---|---|
name | birthday | school_name |
Emily Glass | 25/09/1986 | MIT |
Tom Example | 30/01/1991 | Harvard |
Relationships
One To One
One to one relationship is a "1:1" connection between tables.
from django.db import models
class User(models.Model):
"""User Model"""
name = models.CharField()
birthday = models.CharField()
email = models.CharField()
...
class Profile(models.Model):
"""Profile Model"""
user = models.OneToOneField(User, on_delete=models.CASCADE)
picture = models.ImageField(upload_to='users/pictures', blank=True, null=True)
phone_number = models.CharField()
...
One to Many
One to many relationships is a 1:N table relation.
Example:
from django.db import models
class Picture(models.Model):
"""Picture Model"""
name = models.CharField()
...
class Comments(models.Model):
"""Comments Model"""
picture = models.ForeignKey(Picture, on_delete=models.CASCADE, related_name='comments')
message = models.CharField(max_length=255)
...
Many to Many
Many to many relationship is a N:N table relations.
Example:
from django.db import models
class Pizza(models.Model):
"""Pizza Model"""
size = models.CharField()
...
class Topping(models.Model):
"""Topping Model"""
pizzas = models.ManyToMany(Pizza, on_delete=models.CASCADE, related_name='toppings')
type = models.CharField(max_length=255)
...
or
from django.db import models
class Topping(models.Model):
"""Topping Model"""
type = models.CharField(max_length=255)
...
class Pizza(models.Model):
"""Pizza Model"""
size = models.CharField()
toppings = models.ManyToMany(Topping, on_delete=models.CASCADE, related_name='pizzas')
...