Notes Django
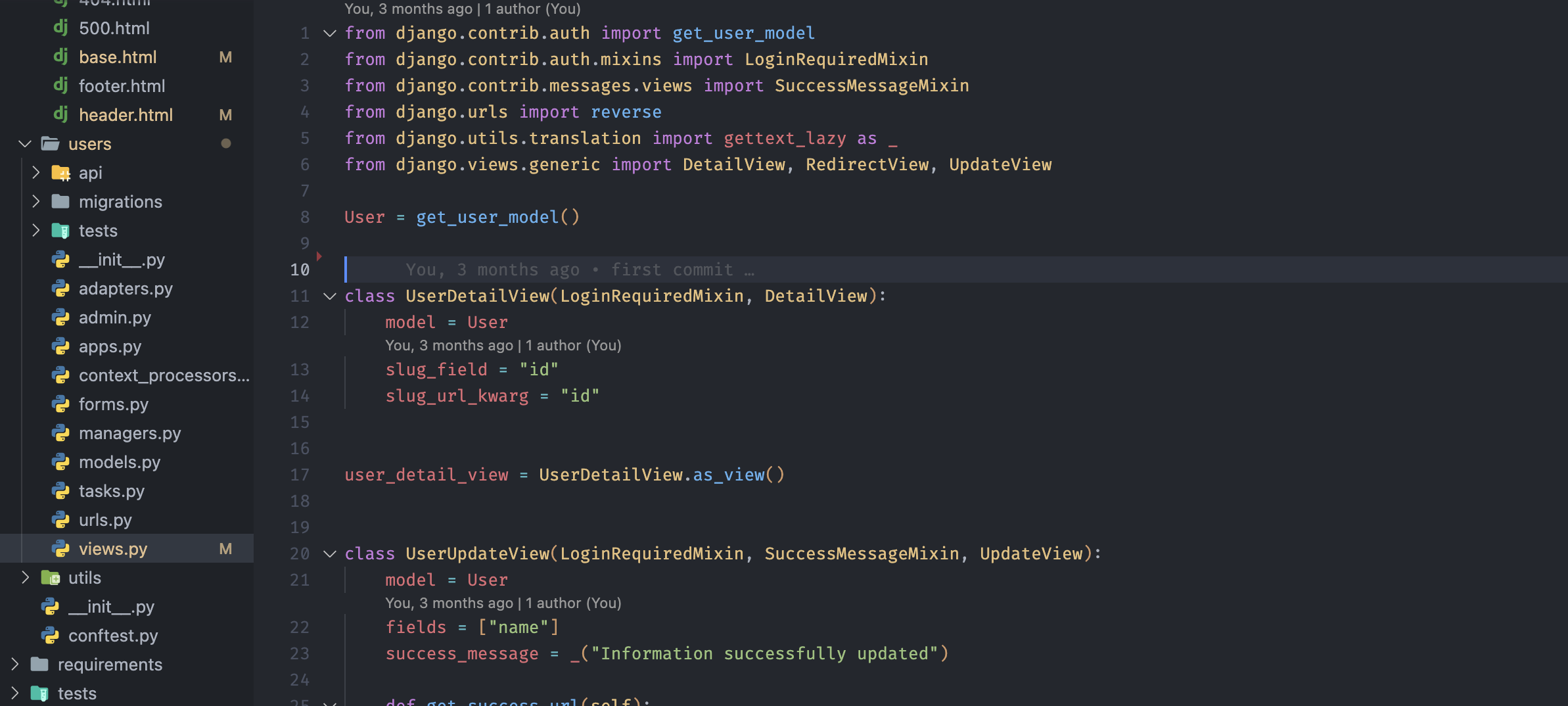
Abstract models
An abstract models is base model a class that could be used as base to create model more complex. But this model is not represent a table in the database. To create an abstract class model I have to create a class Meta inside the class model. E.g
# Django
from django.db import models
class BaseModel(models.Model):
"""Abstract Class"""
created = models.DateTimeField(
'created at',
auto_now_add=True,
help_text='Date time on which the object was created'
)
modified = models.DateTimeField(
'modified at',
auto_now=True,
help_text='Date time on which the object was last modified'
)
class Meta:
""" Meta Obtions """
abstract = True
get_latest_by = 'created'
ordering = ['-created', '-modified']
class Student(BaseModel):
name = models.CharField()
class Meta(BaseModel.Meta):
db_table = 'student_role'
Proxy models
Proxy models allow you to inherit functionality from models. And to add custom functionality. For example:
class Person(models.Model):
first_name = models.CharField()
last_name = models.CharField()
class MyPerson(Person):
class Meta:
proxy = True
def say_something(self, msg):
print(msg)
# good
johan = MyPerson.objects.get(id == 1)
johan.say_something('hi there')
# error
sebas = MyPerson.objects.get(id == 1)
sebas.say_something('hi there')
ViewSets
ViemSet are an complete tool that help to create a CRUD in your API with a few line of code. The ViewSet is create along with a model serializer. This ModelSerializer refer to model which the API will apply the CRUD. Even with all the work made it by a general code, it's possible to customize a special validation in the serializer. To do that it's just change a specific methods. But I got a few Questions.
- How the URLs are index to modified specific instance?
- It's possible to create my own URLs index? 3.