Wine Store
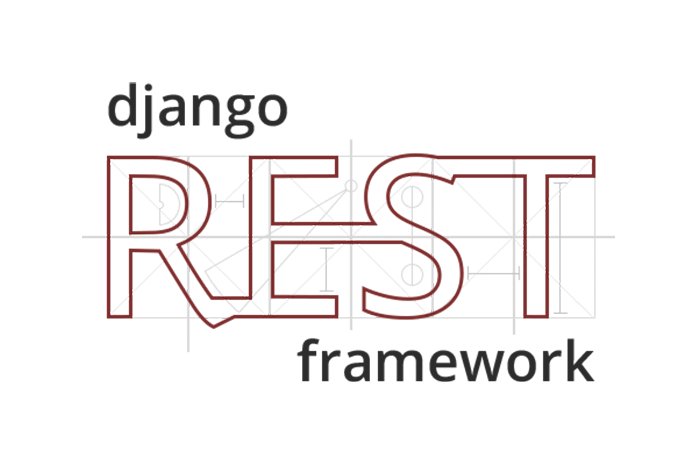
This project is an API for a wine store. It is built with Django and Django Rest Framework. The project implement the basic logic in a e-commerce store (User authentication, User registration, User profile, List and get wines, List and get reviews for a wine, Create, update and delete reviews for a wine, Get, add, update and delete items in a shopping cart, Place an order). The git repository is here
.
This project is 65% complete.
Table of Contents
Overview
The project use cookiecutter-django as starting point. The project use Docker and docker-compose for development and deployment. Also use AllAuth
for and dj_auth_rest
for API authentication.
This is me to work as a monolith application as shown in the next image.
The project use the following technologies: - Django - The web framework used - Django REST Framework - The REST framework used - Docker - The container platform used - PostgreSQL - The database used
The project is divided in apps. The main apps are:
- Cart - This app manage the shopping cart for the users.
- Orders - This app manage the orders for the users.
- Products - This app manage the products (wines) for the users.
- Users - This app manage the users.
- ~~Management~~ - This app manage the admin site. "no implemented yet".
End points
This project has the following end points:
User
- :white_check_mark:
/api/auth/
- dj_rest_auth - Login, Logout, Password change, Password reset, Password reset confirm - :white_check_mark:
/api/auth/registration/
- dj_rest_auth.registration - Register a new user - :white_check_mark:
/api/users/address/
- wine_store.users.api.views.UserAddressViewSet - :white_check_mark:
/api/users/address/<pk>/
- wine_store.users.api.views.UserAddressViewSet - :white_check_mark:
/api/users/payment/
- wine_store.users.api.views.UserPaymentViewSet - :white_check_mark:
/api/users/payment/<pk>/
- wine_store.users.api.views.UserPaymentViewSet
Staff
- :x:
/api/staff/
- List all staff users
Products
- :white_check_mark:
/api/wines/
- wine_store.products.api.views.ProductView - :white_check_mark:
/api/wines/<pk>/
- wine_store.products.api.views.ProductView - :white_check_mark:
/api/wines/<wine_pk>/reviews/
- wine_store.products.api.views.ProductReviewView - :white_check_mark:
/api/wines/<wine_pk>/reviews/<pk>/
- wine_store.products.api.views.ProductReviewView
Cart
- :white_check_mark:
/api/cart/
- wine_store.cart.api.views.CartViewSet - :white_check_mark:
/api/cart/items/
- wine_store.cart.api.views.CartItemViewSet - :white_check_mark:
/api/cart/items/<pk>/
- wine_store.cart.api.views.CartItemViewSet
Orders
- :white_check_mark:
/api/orders/
- wine_store.orders.api.views.OrderViewSet - :white_check_mark:
/api/orders/<pk>/
- wine_store.orders.api.views.OrderViewSet
Settings
To run this project locally (develop mode) you should follow the next steps:
git clone git@github.com:jsuarez-dev/api-wine-store.git
cd api-wine-store
docker-compose -f local.yaml --build
This process will take a while. After that you should run the migrations:
docker-compose -f local.yaml run --rm django python manage.py migrate
Then you can create a superuser:
docker-compose -f local.yaml run --rm django python manage.py createsuperuser
then you can add the features to the database:
docker-compose -f local.yaml run --rm django python manage.py loaddata features/products.json
docker-compose -f local.yaml run --rm django python manage.py loaddata features/account.json
docker-compose -f local.yaml run --rm django python manage.py loaddata features/users.json
This will give you user and data to test the API.
Finally you can run the server:
docker-compose -f local.yaml up -d
Testing
I create a functional test for the API. You can run the test with the following command:
python3 api_functional_test/main.py
Acknowledgements
kaggle wine
- The dataset used for the winesmockaroo
- The dataset used for the usersDjango
cookiecutter-django
AllAuth
dj_auth_rest
Django REST Framework
Docker